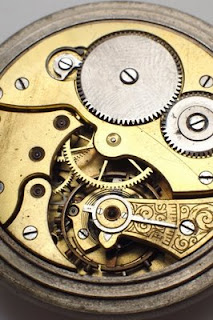
Problems cannot be solved from the same level of consciousness that created it. - Albert EinsteinI was trying to find this famous quote from Einstein and realized that like software, it's actually available in many versions. I could not be sure about the original so in the end just chose one of the shortest.
Sometimes the obvious solutions are unable to solve the underlying problems, because from our level of understanding, we could only see the symptoms and not the cause. Just as an example, Socialism and Communism seem to be a very obvious solution to solve the poverty issue. "Collect money from those who have plenty and distribute among others who don't" - seems to be an obvious solution. However, we could all agree, it does not work as expected.
Basically, I am trying to relate this whole stuff of "level", "abstraction" & "consciousness" etc. with "how should we go about understanding basic software concepts and solve software problems".
Advent of powerful tools, IDEs, libraries and in general this "with a click of mouse" wizards, have made software developer and administrator's life much easier than before. In fact, learning new technology and developing applications has become easier than before, even for the beginners. However, the downside is that most of the developers no longer understand the basic concepts hiding behind the layers of abstraction. This lack of understanding does affect their confidence, while designing or programming, but the problem is more obvious during troubleshooting.
The order of learning may not be important here. e.g. you can first learn about a code generator wizard/IDE and then dig deeper in the layers underneath or learn the basics without using any code generator and then relate it with the generated code.
For example: if you never had an exposure to web related programming, it's better to understand the basics before jumping the gun. There is nothing that will stop anyone from learning ABAP BSPs / WebDynpros / JSPs / Web Services etc., directly. However, you should understand that these are another layers over the basic concepts like : HTML, XML, CSS, core language (e.g. ABAP / Java ) in relation with protocols like HTTP / SOAP. So it's important to understand the underlying concepts first.
What is HTTP Protocol and how can you enhance your understanding by avoiding the abstraction layers?
From Wikipidea : HTTP is a request/response standard of a client and a server. A client is the end-user(software program), the server is the web site. The client making a HTTP request—using a web browser, spider, or other end-user tool—is referred to as the user agent. The responding server—which stores or creates resources such as HTML files and images—is called the origin server. In between the user agent and origin server may be several intermediaries, such as proxies, gateways, and tunnels.Working with the command prompt is a better way to understand the basic concepts. You can try to emulate a basic web-browser (request/response) from the command prompt using telnet.
e.g. to request the first page of Google search html page:
- Start command prompt on your PC using Start->Run -> cmd
- telnet www.google.co.uk 80 [ This is the command to use telnet connection with google at port 80 (web server port)] - press enter
- GET http://www.google.co.uk/ [ This will use the HTTP GET to fetch the default page from google. similarly you can specify any URL on google.co.uk site ] - press enter
- You can see the raw html code as Response from Google web server.
This is to understand the basic browser functionality and how it works. Also, if you are calling a web URL using methods of ABAP Class CL_HTTP_CLIENT then just remember that this class is an abstraction and provides similar functionality as a Browser. And similar to Browser settings, you may need to provide your corporate proxy server information to get this working.
A quick adaptation of the standard SAP example program, for getting the first page from www.google.co.uk is as below:
REPORT Z_RAM_HTTP_CLIENT. * data declarations data: client type ref to if_http_client. data: host type string value 'www.google.co.uk', service type string value '80', path type string value '/', errortext type string, "used for error handling. TIMEOUT type i, subrc like sy-subrc. call method cl_http_client=>create EXPORTING host = host "e.g. www.google.co.uk service = service "port 80 proxy_host = 'proxy.company.com' "proxy server proxy_service = '80' scheme = '1' "HTTP = 1 IMPORTING client = client EXCEPTIONS argument_not_found = 1 internal_error = 2 plugin_not_active = 3 others = 4. if sy-subrc <> 0. write: / 'Client Create failed, subrc = ', sy-subrc. exit. endif. * set http method GET call method client->request->set_method( if_http_request=>co_request_method_get ). * set protocol version client->request->set_version( if_http_request=>co_protocol_version_1_0 ). "protocol = 'HTTP/1.0'. * set request uri (/[? ]) cl_http_utility=>set_request_uri( request = client->request uri = path ). * Send call method client->send EXPORTING timeout = timeout EXCEPTIONS http_communication_failure = 1 http_invalid_state = 2 http_processing_failed = 3 others = 4. if sy-subrc <> 0. call method client->get_last_error IMPORTING code = subrc message = errortext. write: / 'communication_error( send )', / 'code: ', subrc, 'message: ', errortext. exit. endif. * receive call method client->receive EXCEPTIONS http_communication_failure = 1 http_invalid_state = 2 http_processing_failed = 3 others = 4. if sy-subrc <> 0. call method client->get_last_error IMPORTING code = subrc message = errortext. write: / 'communication_error( receive )', / 'code: ', subrc, 'message: ', errortext. exit. endif. * Write data data: data type string. data: fields type tihttpnvp, fields_wa type ihttpnvp. call method client->response->get_header_fields CHANGING fields = fields. loop at fields into fields_wa. write: / 'header_name', fields_wa-name, 'header_value', fields_wa-value. endloop. data = client->response->get_cdata( ). data: line(72) type c, len type i, llen type i. len = strlen( data ). while len > 0. line = data+llen(*). write: / line. len = len - 72. llen = llen + 72. endwhile. if llen < len. line = data+llen(*). write: / line. endif. * close call method client->close EXCEPTIONS http_invalid_state = 1 others = 2.
Further, if you want to understand underlying concepts behind consuming web services ( SOAP over HTTP ) in ABAP then read this excellent Blog Consuming Web Service from ABAP by Durairaj Athavan Raja. This Blog was written by Raja when web service wizard was not available for that release. Even today, this Blog can be used to avoid the wizards and understand the underlying operations of SOAP over HTTP in ABAP.
I am tempted to provide corresponding example from the web service wizard but will try later.
Also, another area I wanted to touch :
Importance of knowing basics like HTML, XML, CSS etc. and how it adds to your understanding while learning dynamic web page generators like BSPs etc. Mainly about BSP/HTMLB extensions and how these extensions generate underlying HTML codes.
For example: HTMLB extension to generate HTML code for a 'Button' is contained in methods DO_AT_BEGINNING, DO_AT_END & RENDER_DEFAULT_START of class CL_HTMLB_BUTTON. Check these methods to have an idea about how different browsers or design type selection (e.g. Classic, Design2002 or Design2003) affect the code generation.
Just to clarify, the suggestion here is to avoid layers, wizards etc. while you are trying to learn the basics. However, use of standard libraries, IDEs and Wizards is recommended for writing productive software as it ensures better productivity, standards, harmony and best practices.
Recommended Reads on related topic:Your feedback is appreciated. However, if you have any technical query on related concepts, please ask your questions in SDN Forums or learn more through SAP Help and Google.
- Don't Reinvent The Wheel, Unless You Plan on Learning More About Wheels By Jeff Atwood
- The Law of Leaky Abstractions By Joel Spolsky
No comments:
Post a Comment
Info : Comments are reviewed before they are published. SPAMs are rejected.